This week we've released version 0.4 of our JavaScript focused code editor. You can read about the background of Scripted here.
The full release notes for 0.4 are here but in this article I'll call out a few of the more interesting changes.
Tooltips
Scripted uses an inferencing engine to build an understanding of your JavaScript code. Scripted 0.3 provided some basic tooltips showing inferred information about function calls. In Scripted 0.4 this has been taken further - not only better formatting but also any jsdoc discovered is now included in the tooltips. Here you can see the tooltip that will appear when you hover over the function call:

Templates
Template support has been enhanced and you can now replace selections with text expansions that embed the original selection. In the first picture we have selected a function call and pressed
Ctrl/Cmd+Space
:
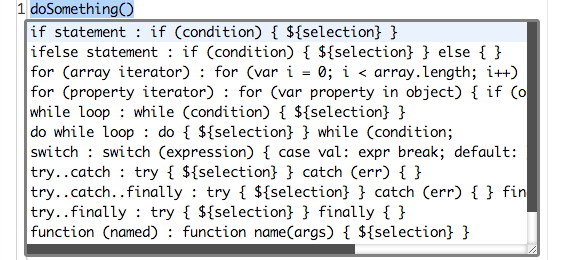
And on selecting the first template completion the editor contents become:

Extensibility
This version of Scripted includes a basic plugin mechanism. It is possible to write just a single .js file, drop it into the right place, and it will extend the behaviour of Scripted. The plugin API is definitely a work-in-progress but you can already achieve some useful functionality. For example we have on-save source transformer plugins that perform operations like removing white space and adding copyright messages. There is more information on the plugin system in the
release notes and
here in the wiki. Basically plugin development involves writing an AMD module, 'require'ing the API pieces and you are good to go.
One of the key use cases we had in mind was enabling you to write a plugin that contributed new annotations to the editor (that appear in the left hand ruler and allow styling of the editor text) . Here is a very simple plugin. This simply locates the names of fruits in your code and adds annotations for them. Perhaps not the most useful plugin but it should show what the key parts of a plugin are…